In the fast-paced world of software development, writing efficient and optimized code is crucial for performance and scalability. C, a powerful and versatile programming language, offers numerous opportunities for optimization. Whether you’re developing embedded systems or high-performance applications, mastering code optimization techniques in C can significantly enhance execution speed and resource management.
Optimization in C isn’t just about making code run faster—it’s about making it more efficient without sacrificing readability or maintainability. Developers often face the challenge of balancing these aspects, especially when dealing with complex algorithms and large codebases. By employing strategic optimization techniques, programmers can ensure their C applications are both robust and agile.
Understanding the nuances of code optimization in C involves exploring various techniques, from loop unrolling to memory management. Each method offers unique advantages, helping developers create applications that are not only faster but also more resource-efficient. This article delves into these techniques, providing insights into crafting high-performance C code.
Code Optimization Techniques in C
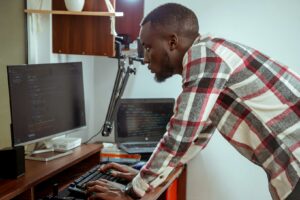
Code optimization in C involves refining a program to improve its performance and resource usage without altering its output. This process focuses on minimizing execution time and memory usage to achieve faster execution and reduced overhead. It ensures that the same functionality is maintained while enhancing the overall efficiency of code.
Levels of Code Optimization
- High-Level Optimization: This involves improving algorithms and data structures. Efficient algorithms and optimized data structures like hash tables and balanced trees can drastically enhance performance.
- Mid-Level Optimization: It focuses on compiler-driven improvements. Developers can leverage compiler flags and settings like -O2 or -O3 to enable optimizations such as function inlining and loop transformations.
- Low-Level Optimization: This involves hardware-specific adjustments. Techniques include using specific CPU instructions or managing cache efficiently to leverage hardware capabilities.
Techniques for Effective Optimization
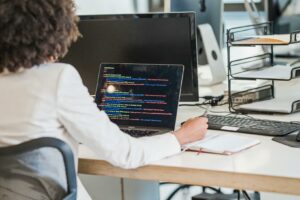
- Loop Unrolling: Reducing the overhead of loop control by expanding the loop body. This increases instruction throughput but might increase code size.
- Memory Management: Efficient use of memory allocators and aligning data can reduce fragmentation and improve cache performance.
- Inlining Functions: Replacing function calls with actual code to reduce overhead, though it may increase executable size.
Tools and Profilers
Tools like gprof and Valgrind help identify performance bottlenecks. Profilers provide data on CPU usage and memory access patterns, guiding developers toward targeted optimizations.
Effective code optimization in C requires a strategic approach to improve performance while preserving code maintainability.
Common Optimization Techniques
In C programming, code optimization enhances performance and resource efficiency. Here are common techniques used to achieve this goal.
Loop Unrolling
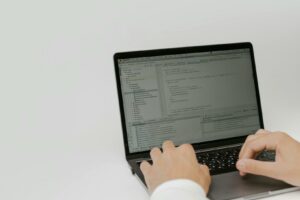
Loop unrolling reduces loop overhead by executing multiple iterations within a single loop iteration. For example, instead of processing one element per iteration, the loop processes several. This approach decreases the number of loop control instructions, improving throughput. However, it’s essential to balance unrolling with code size to avoid negatively impacting cache performance.
Inline Functions
Inlining functions replaces function calls with the function’s body, eliminating call overhead. This technique speeds up execution, especially for small, frequently called functions. Inlining is automatically managed by compilers, but developers can use the inline keyword to suggest it. Overuse increases code size, so it’s crucial to apply it judiciously.
Dead Code Elimination
Dead code elimination removes code segments that don’t affect program results. By analyzing and deleting these sections during compilation, developers clean the code and reduce executables. Compilers like GCC perform this optimization, leading to leaner, faster applications. It ensures that only necessary code contributes to execution, enhancing both speed and resource use.
Quality and Maintainability
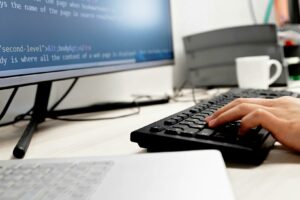
Mastering code optimization techniques in C is crucial for developers aiming to build high-performance applications. By strategically applying methods like loop unrolling, inlining, and effective memory management, they can significantly enhance execution speed and resource efficiency. Profiling tools such as gprof and Valgrind prove invaluable in pinpointing bottlenecks, allowing for precise improvements. Additionally, leveraging compiler optimization flags offers further opportunities to fine-tune performance. Ultimately, the key lies in balancing optimization with maintainability, ensuring that code remains readable and robust while achieving desired efficiency gains. With these insights, developers are well-equipped to tackle the complexities of modern software development in C.